
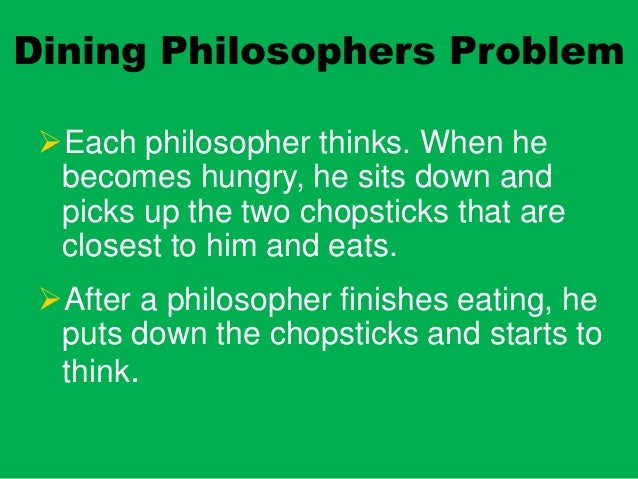
In this case the letters in A-Z get converted to the letters in a-z. It says to take the output from the command on its left (the "cat") and use it as input to the command on its right (the "tr"). Here there is only one file, so it reads and outputs it. It concatenates all files that follow the command and writes the concatenation to the standard output. I don't expect you to understand the commands, cat USConstitution.txt | tr 'A-Z' 'a-z' | tr -cs 'a-z' '\n' | sort | uniq | comm -23 - dictionary.txt For the pipeline to flow smoothly, the production/consumption has to be synchronized.Ī powerful example of this is approach the "pipe" construct in Unix. The Consumers of one item can in turn be Producers of another item, leading to a pipeline through which items flow. The Consumers can't consume until the Producers have produced, and the Producers can't get too big a backlog of unconsumed items. In a "producer/consumer" set-up, one or more Producers output items and one or more Consumers input the items that the Producers produce. Let's look at another example of when concurrent threads need to be synchronized. What could go wrong if it weren't? Producer/consumer Look back at the chat server from last time, and you'll see that access to the list of communicators is synchronized. The other threads wait for the lock to come available. Only one thread can hold the lock at a time, and it gives it up when it returns from the synchronized method. Java implements this by providing a lock for each object. One of them will be allowed to proceed once the first thread leaves the critical region (the synchronized method). If several threads make method calls one is allowed to proceed and the others must wait. The basic idea of a monitor is that only one thread can be inside of it at a time. This increases the amount of concurrency allowed, but can cause problems when a programmer fails to realize that certain operations cannot be safely performed simultaneously. Java allows more flexibility, in that it allows only some methods in the class to be synchronized. If every method in a class is synchronized (except constructors, which cannot and do not need to be synchronized) then we would have a standard monitor.
#Dining philosophers problem java deadlock code
With a monitor there are critical sections of code that only one thread should be allowed to execute at a time. This approach is a version of what is known as a monitor. Thus the increment is "atomic" - it happens without any kind of visible subdivision into pieces that can be interleaved. Only one thread at a time can be executing any synchronized method of a single object. IncrementerTotal.java is a new class that has a synchronized method for incrementing IncrementerSync.java uses it to do a properly synchronized version of two incrementers. All kinds of orders happen, though usually they trample on each other (I/O slows things down and provides a good chance for someone else to slip in).
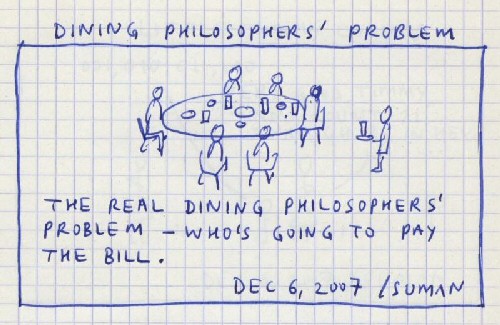
IncrementerInterleaving.java further illustrates this, by putting some print statements in the middle of the incrementing, so that we can see the interleaving. The first thread finishes addition and writes the new total (101), but then so does the second thread. For example, the first thread could get the current total (say 100) and be in the process of adding 1 to it, and meanwhile the second thread also gets the current total (still 100) and begins adding 1 to it. Why isn't it? There can be a low-level interleaving of the thread execution, such that they trample on each other. It looks like the threads should each increment the total a million times, so the total should be 2 million. Otherwise the the main program can print "total at end" before the other two have completed. This method says that the current thread should wait until the thread that join() is called on completes. The main method also calls join() on each of them. The shared resource here is a variable, and each thread is incrementing it. Incrementer.java provides a very simple example of what can happen when two threads are accessing a shared resource. OutlineĪll the code files for today: Consumer.java DiningPhilosophers.java Fork.java Incrementer.java IncrementerInterleaving.java IncrementerSync.java IncrementerTotal.java MessageBox.java MonitoredDiningPhilosophers.java MonitoredFork.java MonitoredPhilosopher.java Philosopher.java Producer.java ProducerConsumer.java For further reading, see the Oracle concurrency tutorial. Today we'll explore related issues in a bit more depth. We saw briefly at the end of last class that we should be careful when concurrent threads are accessing shared resources.
